OverviewObjectivesPart 1: Understanding Flask Blueprints and FormsFlask Blueprints: WTForms and FlaskForm:Part 2: Creating the User ModelPart 3: Implementing CRUD OperationsPart 4: Integrating Flask BlueprintsPart 5: Working with FormsPart 6: Testing and Feedback
Overview
In this assignment, you'll dive deeper into Flask by understanding and implementing Flask Blueprints and utilizing Flask-WTForms for form handling. You'll create a User Model and develop routes/views for basic CRUD (Create, Read, Update, Delete) operations on the user data. Furthermore, you'll explore how to validate data in models and work with forms to capture user input. This assignment is structured to enhance your Flask application structuring skills, focusing on modularity and data handling.
Objectives
- Understand Flask Blueprints: Explore how Blueprints allow you to organize your Flask application into modular components.
- Work with WTForms and FlaskForm: Learn to use these tools for form handling in your Flask application.
- Implement CRUD Operations: Create routes/views to manage user data, including creating, reading, updating, and deleting users.
- Practice Data Validation: Add validations for user data in the models to ensure data integrity.
- Integrate Forms in Views: Modify the view function to handle form data for creating new users.
Part 1: Understanding Flask Blueprints and Forms
Flask Blueprints:
Blueprints are a way to organize a Flask application into smaller and reusable components. They allow you to scale your application and improve its structure by grouping related functionalities. With Blueprints, you can segment your application into distinct features or modules, each capable of operating independently or as part of the whole. This makes development, maintenance, and collaboration on large projects more efficient.
Go through documentation/examples to see how Blueprints are used for structuring applications.
How do Blueprints help in organizing Flask applications?
WTForms and FlaskForm:
WTForms is a library for handling forms in Python, offering a wide variety of field types and validation rules. When used in Flask through the FlaskForm class, it simplifies form creation, data handling, and validation. FlaskForm adds additional functionality specific to Flask applications, including seamless integration with Flask's request object and form rendering capabilities.
Examine how these are implemented in the
forms.py
file for form handling.CSRF Protection
Cross-Site Request Forgery (CSRF) is a security threat where unauthorized commands are transmitted from a user that the web application trusts. WTForms, when used with FlaskForm, provides built-in CSRF protection to safeguard your forms against such attacks. This is achieved by embedding a hidden token in the forms that must match with the server's version to validate the form submission as legitimate.
The Importance of
app.config['SECRET_KEY']
The
app.config['SECRET_KEY']
setting in Flask is essential when using FlaskForm or any form handling that involves CSRF protection. This secret key is used to create a cryptographic token that is used to validate forms and protect against CSRF attacks. The secret key should be a long, random string of bytes, as it is used to sign the CSRF tokens. Without a secret key, Flask applications cannot securely handle forms, making them vulnerable to CSRF and other attacks. Setting a strong SECRET_KEY
is fundamental to the security of your Flask application, ensuring that form data manipulation and CSRF protection are securely managed.What are the benefits of using WTForms for form handling?
Part 2: Creating the User Model
Define a User Model in the
models.py
file with the following attributes:- Name: string
- Age: integer (with a range of 0 to 100)
- Email: string
- Id: Autogenerated
- Gender: Enum (male/female/other)
- PremiumUser: Boolean
Ensure to implement validations for email and age within the model.
Check how validations work in SQLAlchemy using
validates
You can use validations like this inside your model class:
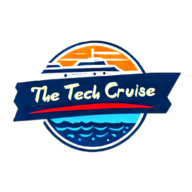
from flask_sqlalchemy import SQLAlchemy
from sqlalchemy.orm import validates
class User(db.Model):
# ... other model definitions
@validates('age')
def validate_age(self, key, age):
if not (0 <= age <= 100):
raise ValueError("Age must be between 0 and 100.")
return age
Instead of jsonifying each value at the return you can have your model handle that as well.
Check how to do that. You can refer the
record
model for that and usage of enum
Part 3: Implementing CRUD Operations
Develop routes/views to perform CRUD operations on the user data:
- get_user: Returns user information based on the ID [Method: GET]
- get_users: Returns all users [Method: GET]
- create_user: Creates a new user [Method: POST]
Test these functionalities using tools like Postman or curl commands.
GET
can be tested directly through the browser.Part 4: Integrating Flask Blueprints
- Create a Blueprint for the user management functionality.
- Register the Blueprint in the
__init__.py
file of the Flask application.
creating a blueprint
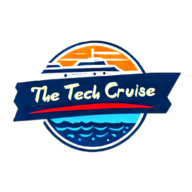
<blueprint_name> = Blueprint(<name>, __name__,template_folder, url_prefix)
registering a blueprint:
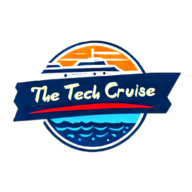
app.register_blueprint(<blueprint_name>)
And the decorator for routes will be changed from
@app.route()
—> @blueprint_name.route()
Check the functionality again after registering the blueprint!
Part 5: Working with Forms
- In the
forms.py
file, create a User Form similar to the existingTimerForm
.
- Modify the
create_user
function to accept input from the newly created form, supporting both GET and POST methods.
Create a template (e.g.,
user_form.html
) for the form, ensuring it's user-friendly and captures all necessary user information. For reference you can check timer_form.html
What roles do
redirect
and url_for
play in form handling?Why is it important for the
create_user
route to accept both POST and GET methods?Here is an example user_form.html
Check different Validators supported by
wtforms.validator
and add the appropriate validators to your form.For radio buttons you might want to use
SelectField
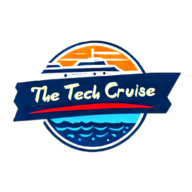
gender = SelectField('Gender', choices=[('male', 'Male'), ('female', 'Female'), ('other', 'Other')], validators=[DataRequired()])
Part 6: Testing and Feedback
- Test your form by creating new users and verify that they appear in the list of all users returned by the
get_users
function.
Notes and Caution
- Ensure your function names are unique to avoid conflicts.
- Be mindful of route paths to prevent overrides.
- Encourage interactivity by posing questions and prompting for feedback.
By the end of this assignment, you'll have a deeper understanding of Flask Blueprints, form handling with WTForms, and implementing CRUD operations in a Flask application. This will be a significant step forward in developing complex and modular Flask applications.