How to integrate the front end with the back end using AxiosStep 1: Install Axios in your front-end projectStep 2: Import Axios in your front-end codeStep 3: Create an Axios instance with the base URL of your back-end APIStep 4: Use the Axios instance to make HTTP requests to the back-end endpointsStep 5: Handle the response and error from the API requestsStep 6: Display or update the data in your front-end UIAbout AuthorsSai Manasa IvaturiSrinivas vaddi
How to integrate the front end with the back end using Axios
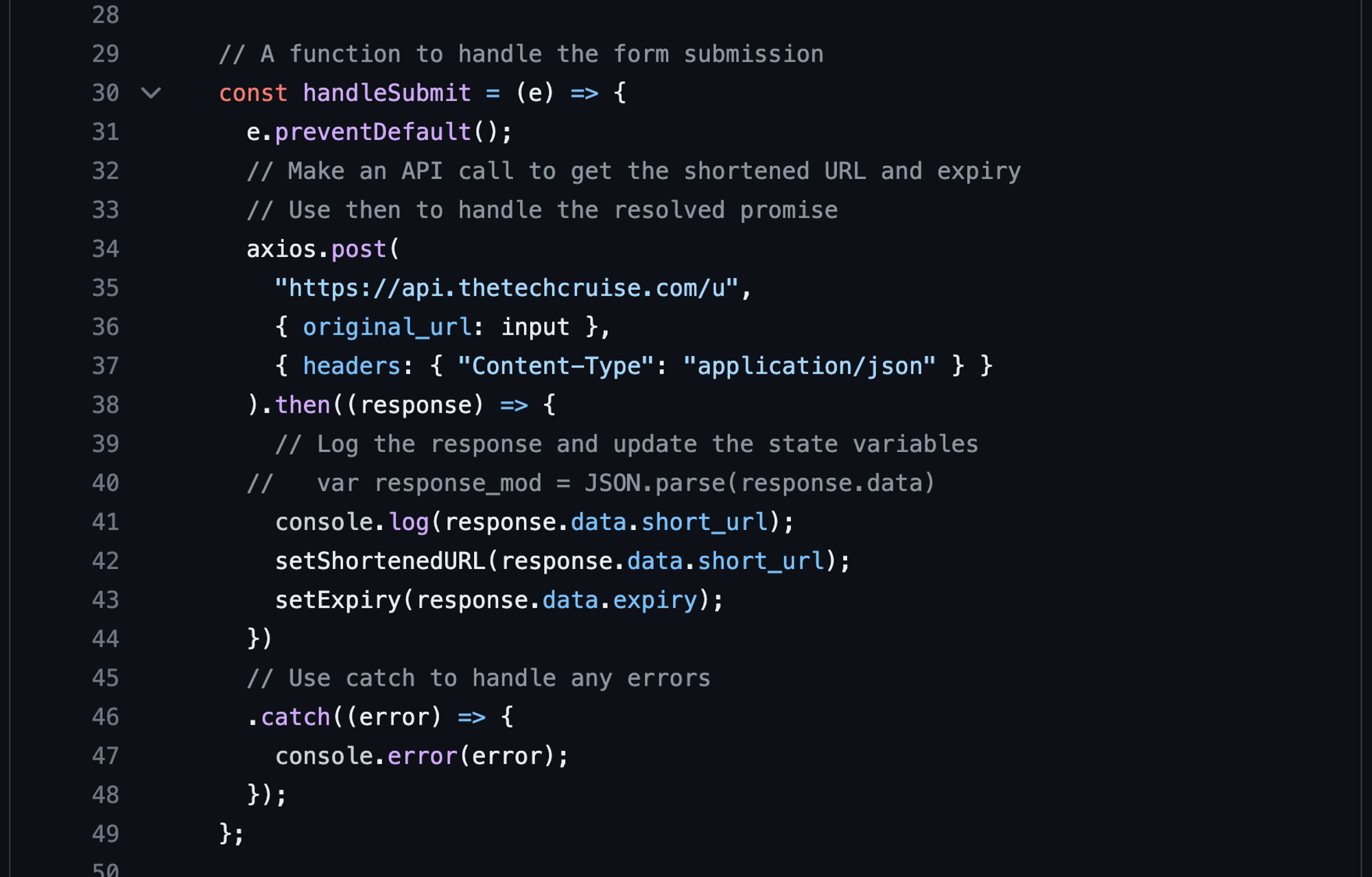
Before you proceed, please please, check if CORS is enabled on your back-end!
Axios is a library that allows you to make HTTP requests from the browser or Node.js. It is a popular choice for working with APIs, which are interfaces that allow different applications to communicate and exchange data. In this tutorial, I will show you how to integrate the front end with the back end using Axios. I will assume that you have a front-end project that uses React and a back-end project that follows the OpenAPI standard.
Step 1: Install Axios in your front-end project
The first step is to install Axios in your front-end project using npm or yarn. This will add Axios as a dependency in your package.json file and allow you to use it in your code. You can run the following commands in your terminal to install Axios:
npm install axios // or yarn add axios
Step 2: Import Axios in your front-end code
The next step is to import Axios in your front-end code where you want to make API requests. You can use the ES6 import syntax to import Axios as a module in your JavaScript files. For example:
import axios from 'axios'
This will make Axios available as a variable in your code.
Step 3: Create an Axios instance with the base URL of your back-end API
The third step is to create an Axios instance with the base URL of your back-end API. You can use the
.create()
method of Axios to create a custom instance of Axios with some default settings. One of the settings you can specify is the base URL, which is the common part of all your API endpoints. For example, if your back-end API is running on http://localhost:3000/api
, you can create an Axios instance named api
with this base URL: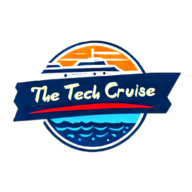
const api = axios.create({ baseURL: 'http://localhost:3000/api' })
Step 4: Use the Axios instance to make HTTP requests to the back-end endpoints
The fourth step is to use the Axios instance to make HTTP requests to the back-end endpoints with the appropriate methods, parameters, headers, and data. You can use the methods of the Axios instance to perform different HTTP operations, such as GET, POST, PUT, DELETE, etc. You can also pass arguments to these methods to specify the parameters, headers, and data for each request. For example, if you want to get a list of users from the
/users
endpoint with a query parameter name=Srinivas
, you can use: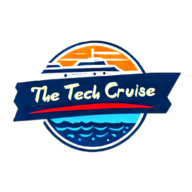
api.get('/users', { params: { name: 'Srinivas' } })
Similarly, if you want to create a new todo item in the
/todos
endpoint with a JSON body containing the title and completed fields, you can use: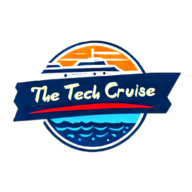
api.post('/todos', { title: 'Learn Axios', completed: false })
Step 5: Handle the response and error from the API requests
The fifth step is to handle the response and error from the API requests using promises or async/await. You can use either promises or async/await syntax to handle the asynchronous nature of HTTP requests. Promises are objects that represent the eventual completion or failure of an asynchronous operation. You can use the
.then()
and .catch()
methods of promises to access the response data or error object from the API requests. For example, if you want to log the response data or error object from the GET request to /users
endpoint, you can use: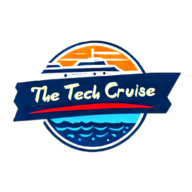
api.get('/users')
.then(response => console.log(response.data))
.catch(error => console.error(error))
Async/await is a syntactic sugar that makes working with promises easier and more readable. You can use the
async
keyword to declare an asynchronous function and the await
keyword to wait for a promise to resolve or reject. You can also use try/catch blocks to handle errors. For example, if you want to do the same thing as the previous example but using async/await syntax, you can use: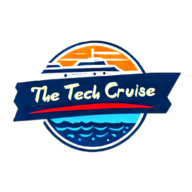
async function getUsers() {
try {
const response = await api.get('/users');
console.log(response.data);
} catch (error) {
console.error(error);
}
}
Step 6: Display or update the data in your front-end UI
The final step is to display or update the data in your front-end UI according to the response or error from the API requests. You can use various techniques and libraries to manage the data flow between your front-end components and your back-end API. For example, you can use React hooks such as useState and useEffect to store and update the data in your component state and render it in your UI. You can also use state management libraries such as Redux or MobX to store and update the data in a global state and connect it to your components. You can also use UI libraries such as Material UI or Bootstrap to style and layout your components.
That’s it! You have learned how to integrate the front end with the back end using Axios. If you want to learn more about Axios, you can check out their official documentation. If you want to see some examples of how to use Axios with React, you can check out this tutorial.
About Authors
Sai Manasa Ivaturi
I'm a Software Development Engineer based in Atlanta, Georgia with 5+ years of experience in the software industry. My focus area has been Backend development and full-stack development.
View my Resume here.
Masters Degree in Computer Science Indiana University, Bloomington
Jan 22 - May 23
Bachelors Degree in Computer Science Pragati Engineering College, India
Aug 14 - April 18
Srinivas vaddi
Hi! I’m a recent master’s graduate from Indiana University Bloomington (IUB) 🎓 and a Software Development Engineer with 4+ years of experience. Looking for #jobs!
My areas of expertise are Software Development, DevOps, Testing, Integration, Data Engineering and Data Analytics. Mostly worked on Python, Django/Flask, Apache Airflow, Apache Spark, AWS, and DevOps. I have a versatile background & a ‘can do’ attitude 🤓.
I like blogging and sharing knowledge. I’ve built a server at home from scratch! I used it to learn various technologies and to contribute to the open-source. I love tech, philosophy, literature, and history. My favorite books 📚 of all time are ‘The Alchemist’ and ‘Chanakya Neeti’ 🙌.
Masters Degree in Computer Science Indiana University, Bloomington Aug 23, 2021 → Dec 17, 2022
Bachelors Degree in Computer Science Gitam University (Deemed to be) Jun 1, 2014 → Apr 1, 2018