Introduction
A contact card is a component that displays some personal or professional information about a person, such as their name, photo, phone number, email, social media links, etc. It can be used to showcase your profile, portfolio, or resume on a website or app.
To create a reusable ReactJS contact card, you will need to use some libraries and tools, such as:
- React, a JavaScript library for building user interfaces.
- Material-UI, a popular UI framework for React that provides ready-made components and icons.
- prop-types, a library for type checking the props passed to your components.
- create-react-app, a tool that sets up a modern React project with no configuration.
Steps
- Create a new React project using
create-react-app
. You can use the commandnpx create-react-app contact-card
in your terminal.
- Install
material-ui
andprop-types
usingnpm install @material-ui/core @material-ui/icons prop-types
in your terminal.
- Create a new file called
ContactCard.js
in thesrc
folder of your project. This is where you will write your contact card component.
- Import the necessary modules and components from
react
,material-ui
, andprop-types
at the top of your file. For example:
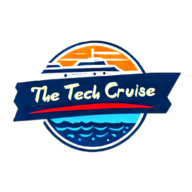
import React from 'react';
import PropTypes from 'prop-types';
import { Card, CardContent, CardMedia, Typography, IconButton } from '@material-ui/core';
import { GitHub, LinkedIn, Mail, Phone, Web } from '@material-ui/icons';
- Define your contact card component as a function that takes some props as parameters. The props are the data that you want to display on your card, such as
image
,firstName
,lastName
,phone
,email
,github
,linkedin
,website
, andbackgroundColor
. For example:
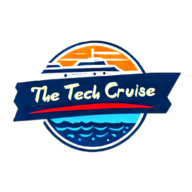
function ContactCard(props) {
// destructure the props object
const { image, firstName, lastName, phone, email, github, linkedin, website, backgroundColor } = props;
// return the JSX code for your component
return (
// ...
);
}
- Use the
<Card>
component from Material-UI to create the main container for your contact card. You can pass thebackgroundColor
prop as a style attribute to customize the background color of your card. For example:
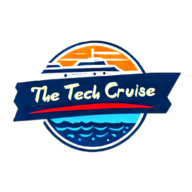
return (
<Card style={{ backgroundColor }}>
// ...
</Card>
);
- Use the
<CardMedia>
component from Material-UI to display the image of the person on your card. You can pass theimage
prop as a source attribute to specify the image URL. You can also use some style attributes to adjust the size and position of the image. For example:
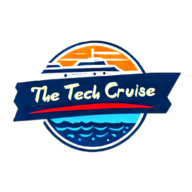
<Card style={{ backgroundColor }}>
<CardMedia
style={{ height: 200 }}
image={image}
title={`${firstName} ${lastName}`}
/>// ...
</Card>
- Use the
<CardContent>
component from Material-UI to create a section for displaying the text and icons on your card. You can use some style attributes to adjust the padding and alignment of the content. For example:
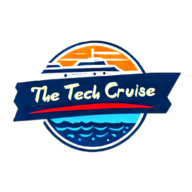
<Card style={{ backgroundColor }}>
<CardMedia
style={{ height: 200 }}
image={image}
title={`${firstName} ${lastName}`}
/><CardContent style={{ padding: 16, textAlign: 'center' }}>
// ...
</CardContent>
</Card>
- Use the
<Typography>
component from Material-UI to display the name of the person on your card. You can pass thefirstName
andlastName
props as children of the component. You can also use some attributes to specify the variant, color, and font weight of the text. For example:
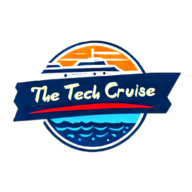
<CardContent style={{ padding: 16, textAlign: 'center' }}>
<Typography variant="h5" color="textPrimary" style={{ fontWeight: 'bold' }}>
{firstName} {lastName}
</Typography>// ...
</CardContent>
- Use the
<IconButton>
component from Material-UI to display the icons for phone, email, GitHub, LinkedIn, and website on your card. You can pass the corresponding props as href attributes to specify the links for each icon. You can also use some attributes to specify the size, color, and edge of each icon button. For example:
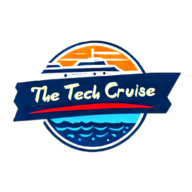
<CardContent style={{ padding: 16, textAlign: 'center' }}>
<Typography variant="h5" color="textPrimary" style={{ fontWeight: 'bold' }}>
{firstName} {lastName}
</Typography><IconButton size="small" color="primary" edge="start" href={phone}>
<Phone />
</IconButton><IconButton size="small" color="primary" href={email}>
<Mail />
</IconButton><IconButton size="small" color="primary" href={github}>
<GitHub />
</IconButton><IconButton size="small" color="primary" href={linkedin}>
<LinkedIn />
</IconButton><IconButton size="small" color="primary" edge="end" href={website}>
<Web />
</IconButton>
</CardContent>
- Use the
PropTypes
module to define the types and default values of the props for your contact card component. This will help you to validate the data passed to your component and avoid errors. For example:
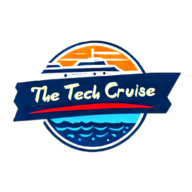
ContactCard.propTypes = {
image: PropTypes.string,
firstName: PropTypes.string,
lastName: PropTypes.string,
phone: PropTypes.string,
email: PropTypes.string,
github: PropTypes.string,
linkedin: PropTypes.string,
website: PropTypes.string,
backgroundColor: PropTypes.string,
};
ContactCard.defaultProps = {
image: '',
firstName: '',
lastName: '',
phone: '',
email: '',
github: '',
linkedin: '',
website: '',
backgroundColor: '#fff',
};
- Export your contact card component as a default export from your file. This will allow you to import and use it in other files. For example:
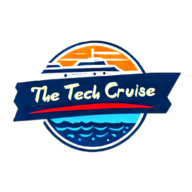
export default ContactCard;
- Create a new file called
App.js
in thesrc
folder of your project. This is where you will use your contact card component and pass some data as props.
- Import the
React
module and yourContactCard
component at the top of your file. For example:
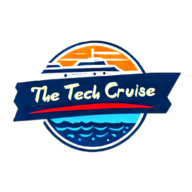
- Define your app component as a function that returns some JSX code. You can use the
<ContactCard>
component and pass some data as props to display a contact card on your app. For example:
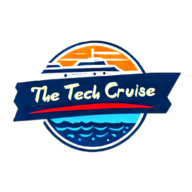
function App() {
return (
<div style={{ display: 'flex', justifyContent: 'center', alignItems: 'center', height: '100vh' }}>
<ContactCard
image="https://avatars.githubusercontent.com/u/69631?v=4"
firstName="Dan"
lastName="Abramov"
phone="+1-234-567-8901"
email="[email protected]"
github="https://github.com/gaearon"
linkedin="https://www.linkedin.com/in/dan-abramov-25a55a5/"
website="https://overreacted.io/"
backgroundColor="#e0f2f1"
/>
</div>
);
}
- Export your app component as a default export from your file. This will allow you to render it in the root element of your HTML document. For example:
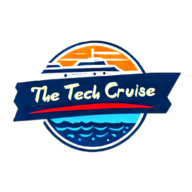
- Run your app using
npm start
in your terminal. You should see a contact card displayed on your browser window.
Β
Congratulations! You have successfully created a reusable ReactJS contact card. π
Β
I hope this tutorial was helpful and informative for you. If you have any questions or feedback, please let me know. Iβm always happy to chat with you. π
References
About Authors
Sai Manasa Ivaturi
I'm a Software Development Engineer based in Atlanta, Georgia with 5+ years of experience in the software industry. My focus area has been Backend development and full-stack development.
View my Resume here.
Masters Degree in Computer Science Indiana University, Bloomington
Jan 22 - May 23
Bachelors Degree in Computer Science Pragati Engineering College, India
Aug 14 - April 18
Srinivas vaddi
Hi! Iβm a recent masterβs graduate from Indiana University Bloomington (IUB) π and a Software Development Engineer with 4+ years of experience. Looking for #jobs!
My areas of expertise are Software Development, DevOps, Testing, Integration, Data Engineering and Data Analytics. Mostly worked on Python, Django/Flask, Apache Airflow, Apache Spark, AWS, and DevOps. I have a versatile background & a βcan doβ attitude π€.
I like blogging and sharing knowledge. Iβve built a server at home from scratch! I used it to learn various technologies and to contribute to the open-source. I love tech, philosophy, literature, and history. My favorite books π of all time are βThe Alchemistβ and βChanakya Neetiβ π.
Masters Degree in Computer Science Indiana University, Bloomington Aug 23, 2021 β Dec 17, 2022
Bachelors Degree in Computer Science Gitam University (Deemed to be) Jun 1, 2014 β Apr 1, 2018