Thoughts and Talk
When we approach problem-solving in programming, it's often tempting to dive straight into using various data structures or algorithms, each with its unique strengths and purposes. Indeed, these tools are vital in crafting efficient and elegant solutions. Yet, it's crucial to remember that not every problem demands such complexity.
At its heart, problem-solving is a common thread shared between mathematics, science, and programming. In many cases, programming serves merely as a tool—a means to execute well-established mathematical or scientific principles. For instance, a brute-force approach or complex backtracking algorithms might initially seem necessary to solve a given problem, but often, a deeper understanding of fundamental concepts like averages can provide a simpler and more straightforward solution.
This perspective reminds us that before we jump into coding, pausing to consider the underlying principles can lead to more insightful and efficient solutions. It's about seeing beyond the immediate programming challenge and appreciating the mathematical beauty that underpins it. Such an approach not only simplifies the solution but also enriches our appreciation of the problem-solving process, blending logic with creativity in a distinctly human way.
And that is the TL;DR of how this problem can be solved.
Problem Statement
Given an array of integers representing dice rolls and an integer representing the target mean for all dice rolls, including those missing, the task is to determine the values of the missing rolls. The number of missing rolls is given by
n
, and each roll can result in a value from 1 to 6. The goal is to ensure the average of all rolls (both provided and missing) equals the target mean.Hints
- Dice Value Range: All roll values must be between 1 and 6.
- Total Sum Calculation: The total sum required to achieve the target mean must consider both existing and missing rolls.
- Adjustment of Missing Rolls: Distribute the required sum across the missing rolls evenly and adjust for any remainder.
Approaches Explored
- Base Calculation Approach
- Pros: Direct computation of the total sum needed and straightforward distribution of values.
- Cons: Requires careful handling of cases where calculated values fall outside the valid dice range.
- Dynamic Adjustment
- Pros: Adjusts the values dynamically to ensure all are within the valid range, utilizing any remainder effectively.
- Cons: This method is slightly more complex to implement, particularly in ensuring that adjustments are correctly made within the range constraints.
Finalized Approach
The chosen approach is grounded in understanding the relationship between the mean, the sum of elements, and the number of observations (dice rolls). It involves:
- Calculating the sum needed from the missing rolls to achieve the target mean.
- Evenly distributing this sum across the missing rolls.
- Adjusting for any remainder to ensure all values remain within the valid range of dice rolls (1 to 6).
Executable Code
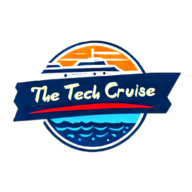
from typing import List
class Solution:
def missingRolls(self, rolls: List[int], mean: int, n: int) -> List[int]:
m = len(rolls)
total_sum_needed = (mean * (m + n) - sum(rolls))
base_value = total_sum_needed // n
result = [base_value] * n
remainder = total_sum_needed % n
if base_value > 6 or base_value < 1 or (remainder and base_value == 6):
return []
for i in range(remainder):
result[i] += 1
return result
# this below part can be ignored this is only in place for you to run.
def main():
# Create an instance of the Solution class
sol = Solution()
# Test case 1
rolls1 = [3, 2, 4, 3]
mean1 = 4
n1 = 2
print("Test Case 1 - Expected Output: [6, 5] or similar with sum = 20")
print("Output:", sol.missingRolls(rolls1, mean1, n1))
# Test case 2
rolls2 = [1, 5, 6]
mean2 = 3
n2 = 4
print("Test Case 2 - Expected Output: Empty list because it's impossible")
print("Output:", sol.missingRolls(rolls2, mean2, n2))
# Test case 3
rolls3 = [1, 2, 3, 4]
mean3 = 6
n3 = 4
print("Test Case 3 - Expected Output: [6, 6, 6, 6] or any combination that fits the criteria")
print("Output:", sol.missingRolls(rolls3, mean3, n3))
if __name__ == "__main__":
main()
Code Explanation
In this section, I'll explain the key components of the executable code provided above, breaking down how each part contributes to solving the problem.
Code Breakdown
- Initialization of Variables:
m
: The number of existing dice rolls.total_sum_needed
: The total sum required from all rolls (existing and missing) to achieve the target mean.base_value
: The initial value for each missing roll, calculated by dividing the total needed sum by the number of missing rolls (n
).result
: An array initialized withn
entries, each set tobase_value
.remainder
: The remainder when the total sum needed is divided byn
, which will be distributed among the rolls to ensure the correct total sum.
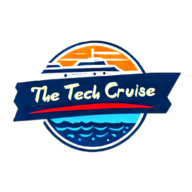
# pyodide: false
m = len(rolls)
total_sum_needed = (mean * (m + n) - sum(rolls))
base_value = total_sum_needed // n
result = [base_value] * n
remainder = total_sum_needed % n
- Validation Check:
- If
base_value
is greater than 6 or less than 1, it's impossible to adjust the rolls to meet the range criteria. - If
base_value
equals 6 and there's still a remainder, it’s impossible to add to any roll without exceeding the maximum dice value of 6.
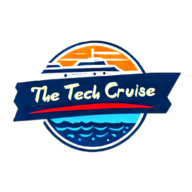
# pyodide: false
if base_value > 6 or base_value < 1 or (remainder and base_value == 6):
return []
This condition ensures that each roll value is within the valid range for a dice (1 to 6):
- Adjusting for the Remainder:
- The remainder is distributed by incrementally increasing the value of each roll starting from the first. This loop runs
remainder
times, adding one to each of the firstremainder
rolls in theresult
array.
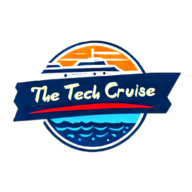
# pyodide: false
for i in range(remainder):
result[i] += 1
Overall Flow
The code first calculates how much total sum is needed from the missing rolls to achieve the desired average across all rolls. It assigns an equal part of this sum to each missing roll and distributes any leftover sum that couldn't be evenly divided. The validation step ensures all resulting roll values are within the acceptable range, and if not, it returns an empty list, indicating no solution under the constraints. This approach efficiently handles the arithmetic distribution and validation within the constraints set by dice roll values.
Discussion on Complexity
- Time Complexity:
O(n)
, as the solution primarily involves initializing and potentially modifying an array of sizen
.
- Space Complexity:
O(n)
, due to the storage requirements for the result list.
This approach not only leverages fundamental arithmetic principles to solve the problem but also incorporates efficient iteration and conditional checks to ensure the validity of the dice values. It strikes a balance between mathematical insight and computational efficiency, addressing the problem requirements with precision.